Fragment
Setup
Add the katalog-androidview
package.
dependencies {
implementation("com.moriatsushi.katalog:katalog:`LATEST_VERSION`")
implementation("com.moriatsushi.katalog:katalog-androidview:`LATEST_VERSION`")
}
Examples
To add a Fragment
, use the fragment
method.
If you omit the name
, the Fragment class name will be used.
res/layout/fragment_sample.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_gravity="center"
android:text="Hello, World"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</FrameLayout>
class SampleFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
val binding = FragmentSampleBinding.inflate(inflater, container, false)
return binding.root
}
}
fragment {
SampleFragment()
}
By default, match_parent
is used for both width and height.
You can change the size of the View by setting the layoutParams
.
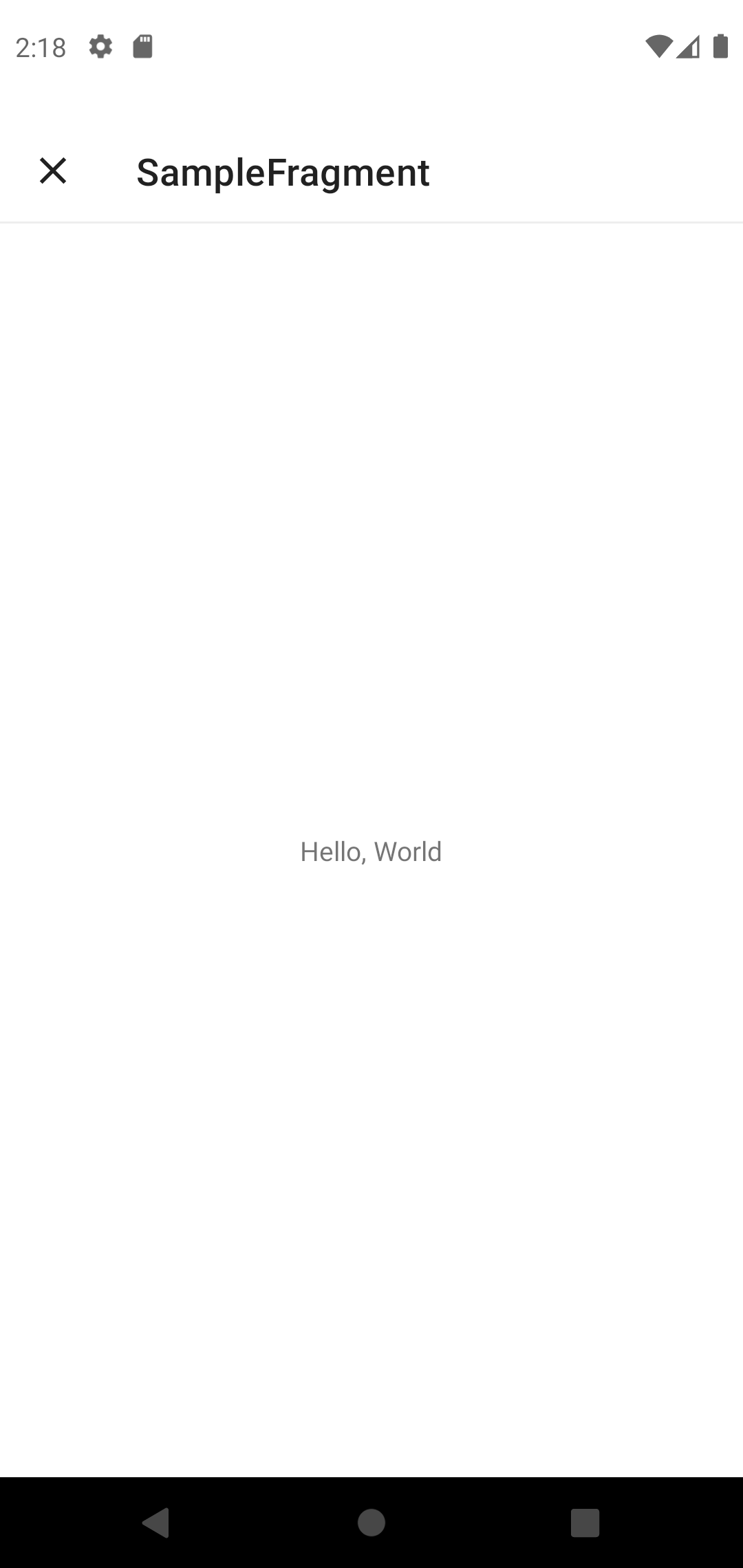
If you want to know when the View is created, use the onCreateView
parameter.
You can update the View safely.
res/layout/fragment_sample.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text"
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</FrameLayout>
class SampleFragment : Fragment() {
private var binding: FragmentSampleBinding? = null
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
binding = FragmentSampleBinding.inflate(inflater, container, false)
return binding!!.root
}
fun updateText(value: String) {
binding!!.text.text = value
}
override fun onDestroyView() {
super.onDestroyView()
binding = null
}
}
fragment(
onCreateView = { fragment ->
fragment.updateText("Updated!")
}
) {
SampleFragment()
}
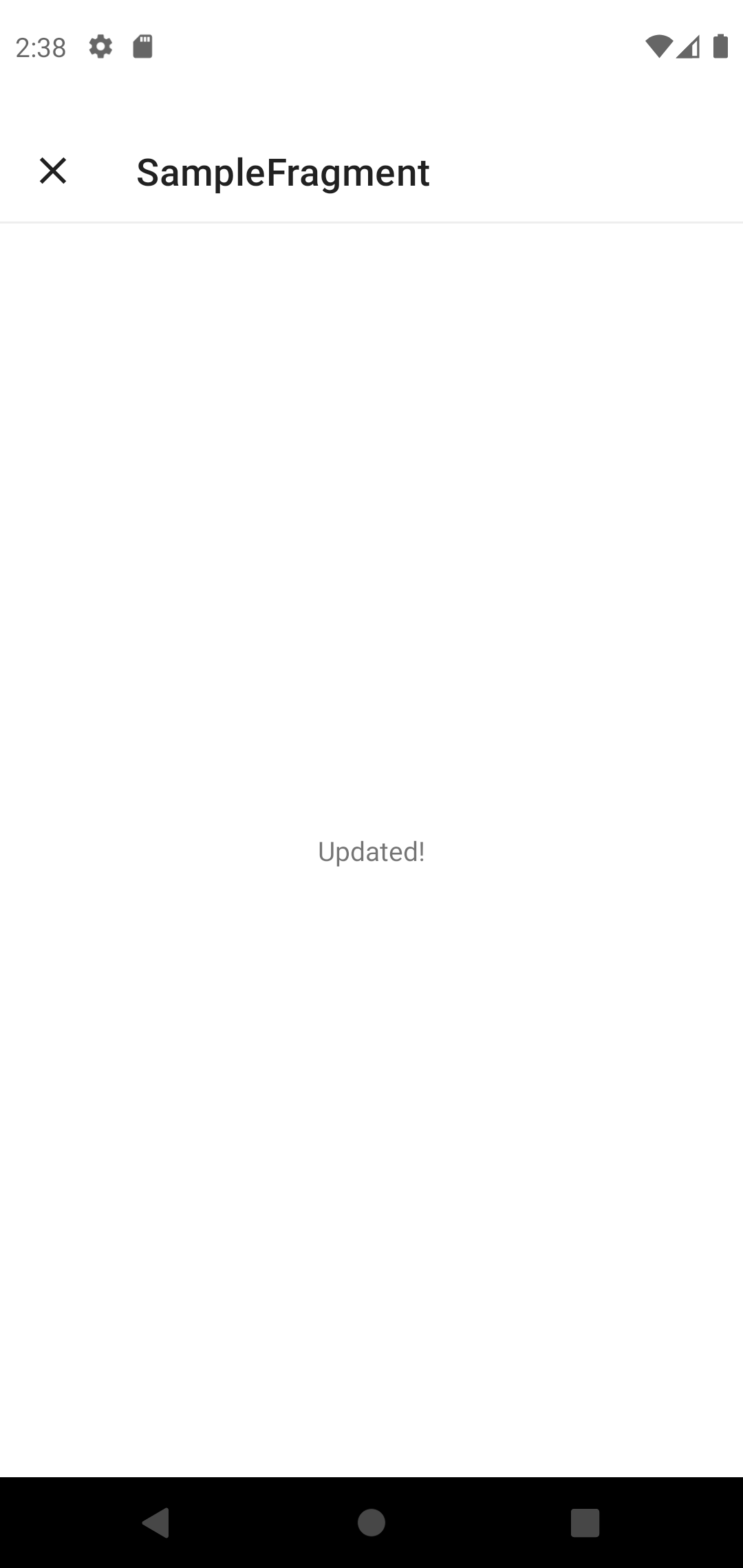
Parameters
name | description |
---|---|
name | The UI Component name. If you omit this, the Fragment class name will be used. |
layoutParams | Specifies the size of the View. By default, match_parent is used for both width and height. |
onCreateView | Called when the Fragment's View is created. This is a safe way to update the View. Inside the lambda expression, you can access activity , context , and lifecycleOwner . This is optional. |
definition | Create a Fragment instance. Inside the lambda expression, you can access activity, context, and lifecycleOwner. |