Android View
Setup
Add the katalog-androidview
package.
dependencies {
implementation("com.moriatsushi.katalog:katalog:`LATEST_VERSION`")
implementation("com.moriatsushi.katalog:katalog-androidview:`LATEST_VERSION`")
}
Examples
To add a Android View
, use the view
method.
Context
is available within the lambda function.
If you omit the name
, the class name will be used.
view(
name = "TextView"
) {
TextView(context).apply {
text = "Hello, World"
}
}
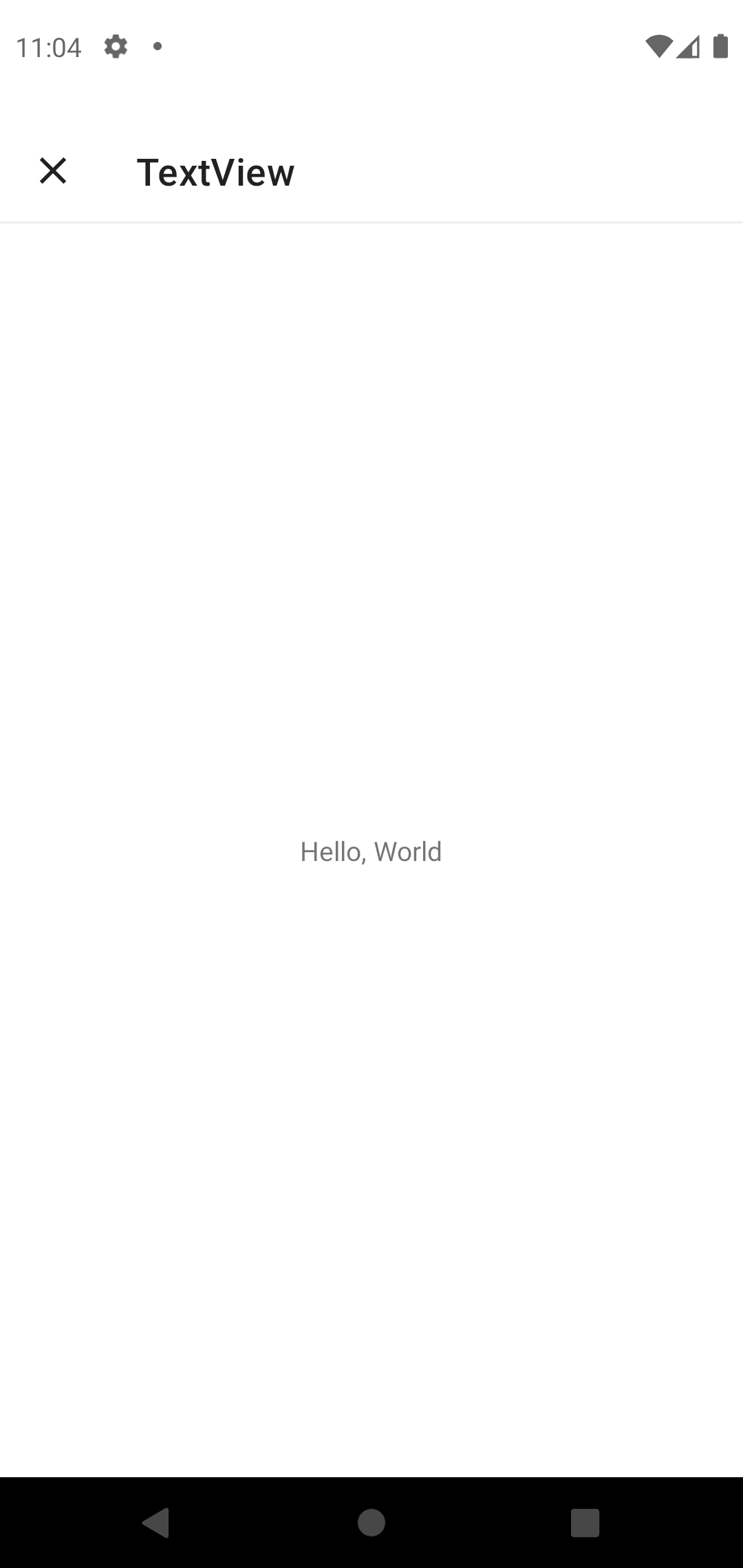
By default, wrap_content
is used for both width and height.
You can change the size of the View by setting the layoutParams
.
view(
name = "TextView",
layoutParams = MATCH_WIDTH_MATCH_HEIGHT
) {
TextView(context).apply {
setBackgroundColor(Color.RED)
text = "Hello, World"
}
}
The following four options are available.
WARP_WIDTH_WRAP_HEIGHT
- It is default.MATCH_WIDTH_WRAP_HEIGHT
WRAP_WIDTH_WRAP_HEIGHT
MATCH_WIDTH_MATCH_HEIGHT
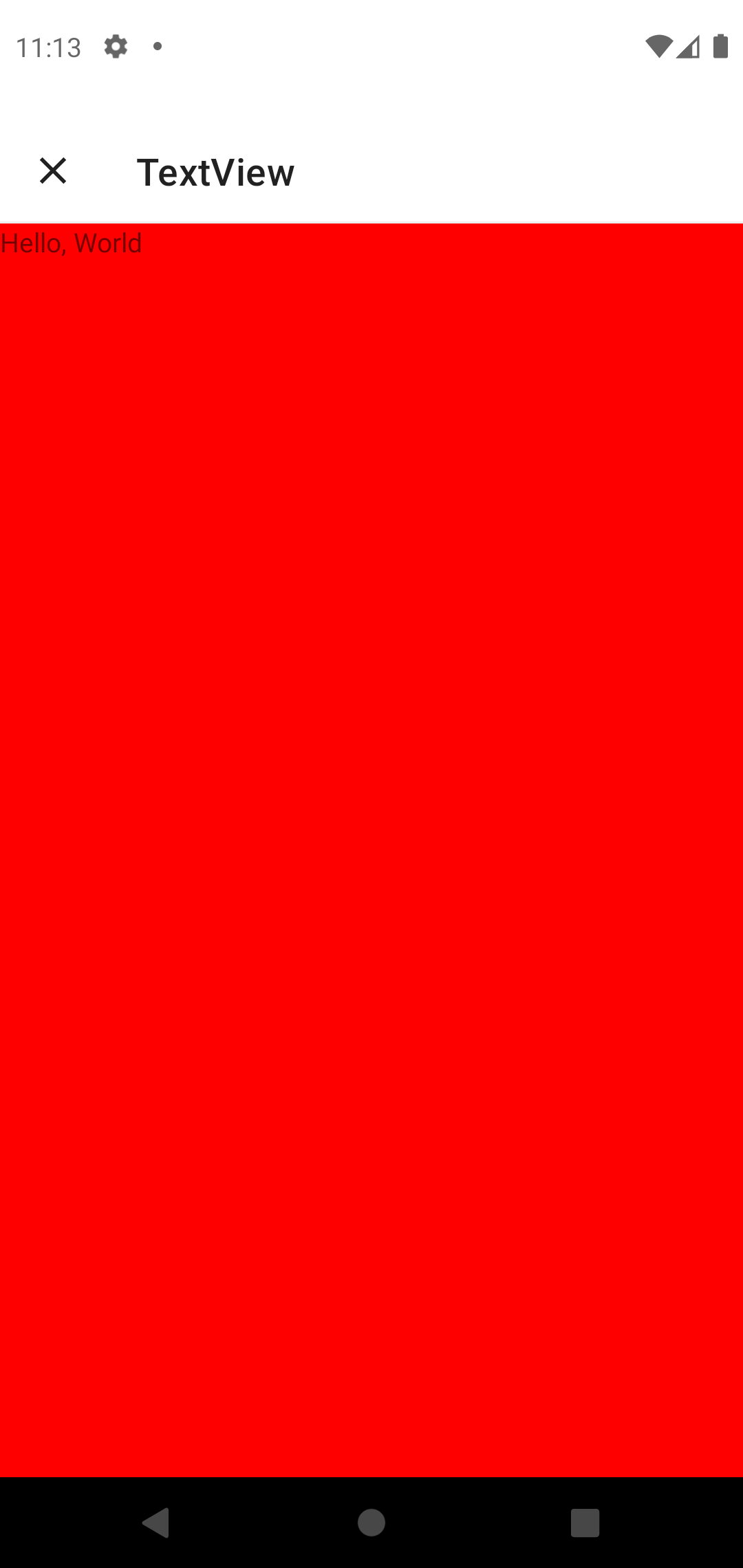
You can also specify a number directly. Note that these values are in pixels.
view(
name = "TextView",
layoutParams = ViewGroup.LayoutParams(600, 300)
) {
TextView(context).apply {
setBackgroundColor(Color.RED)
text = "Hello, World"
}
}
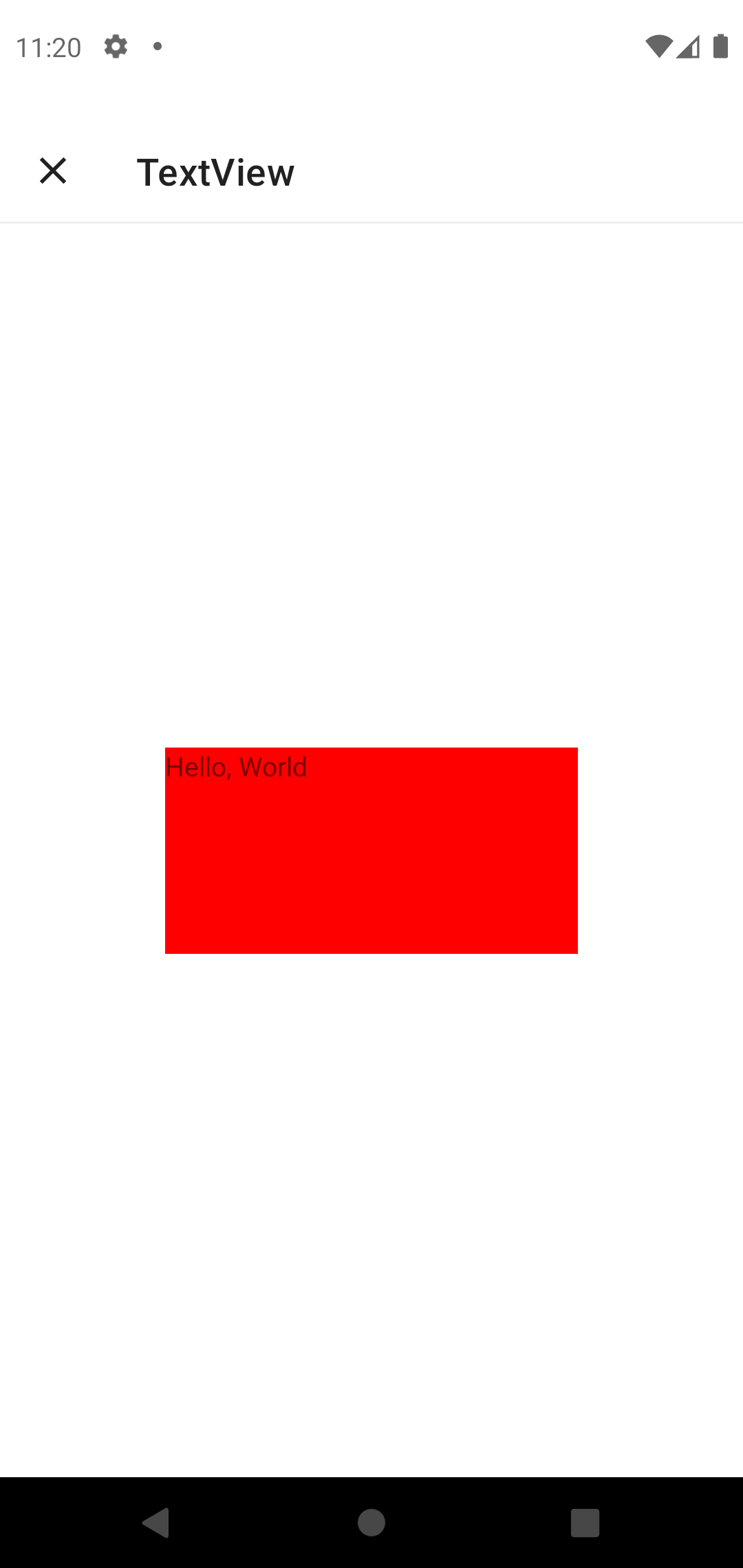
You can use the LifecycleOwner
to observe a LiveData
or Flow
.
view(
name = "Counter"
) {
val liveData = MutableLiveData(0)
val view = TextView(context)
liveData.observe(lifecycleOwner) {
view.text = it.toString()
}
view.setOnClickListener {
liveData.value = (liveData.value!! + 1) % 10
}
view
}
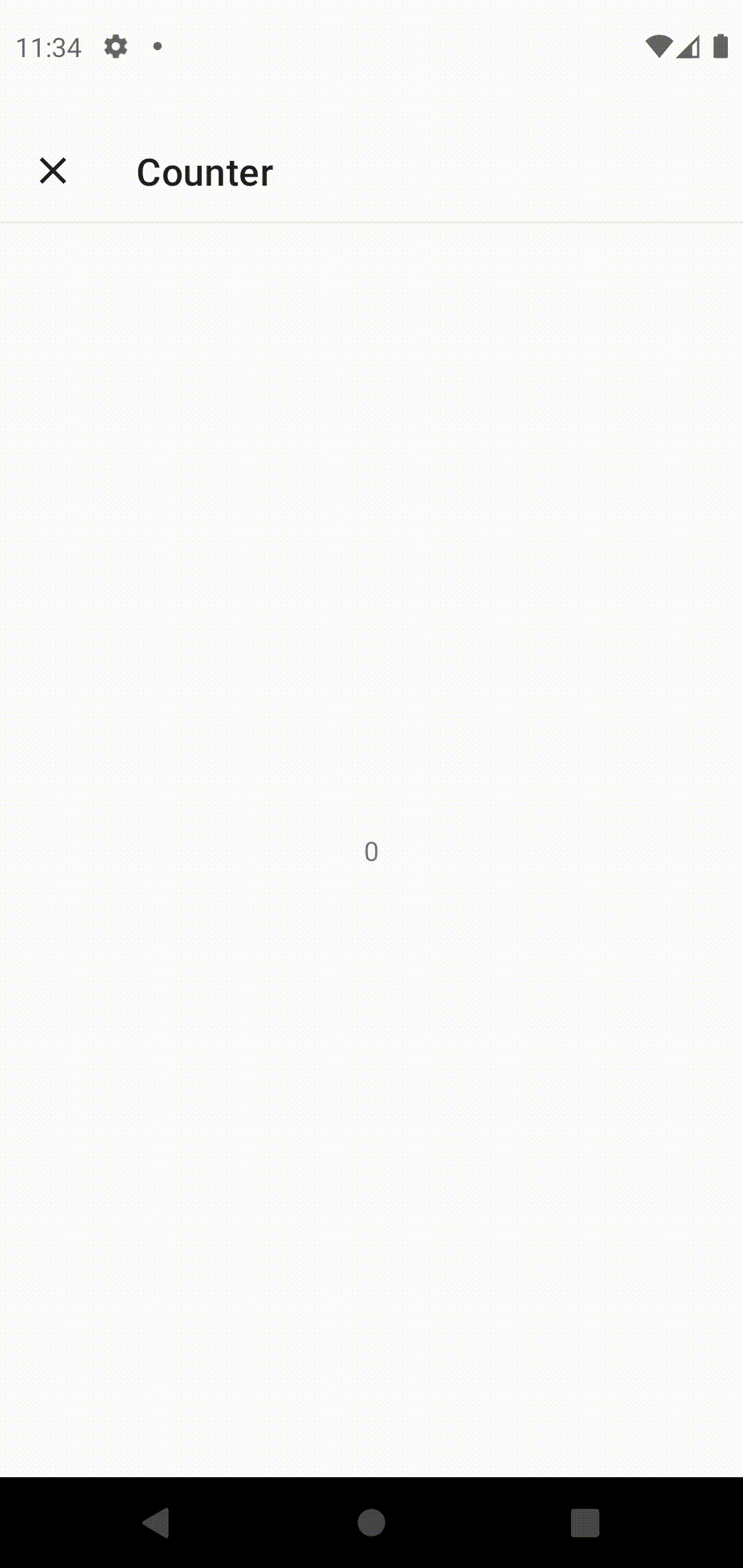
Parameters
name | description |
---|---|
name | The UI Component name. If you omit this, the class name will be used. |
layoutParams | Specifies the size of the View. By default, wrap_content is used for both width and height. |
definition | Create an instance of View. Inside the lambda expression, you can access activity , context , and lifecycleOwner . |