Getting Started
step1: Add the dependency
Add Maven Central repository to your build.gradle
.
repositories {
mavenCentral()
}
Add the package dependencies to your build.gradle
.
dependencies {
implementation("com.moriatsushi.katalog:katalog:1.2.2")
}
step2: Register the UI component
Just run the registerKatalog
function in your application.
To register a Composable
, use the compose
function.
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
registerKatalog(
title = "My App Catalog"
) {
compose("UI Component") {
Text(text = "Hello, World")
}
}
}
}
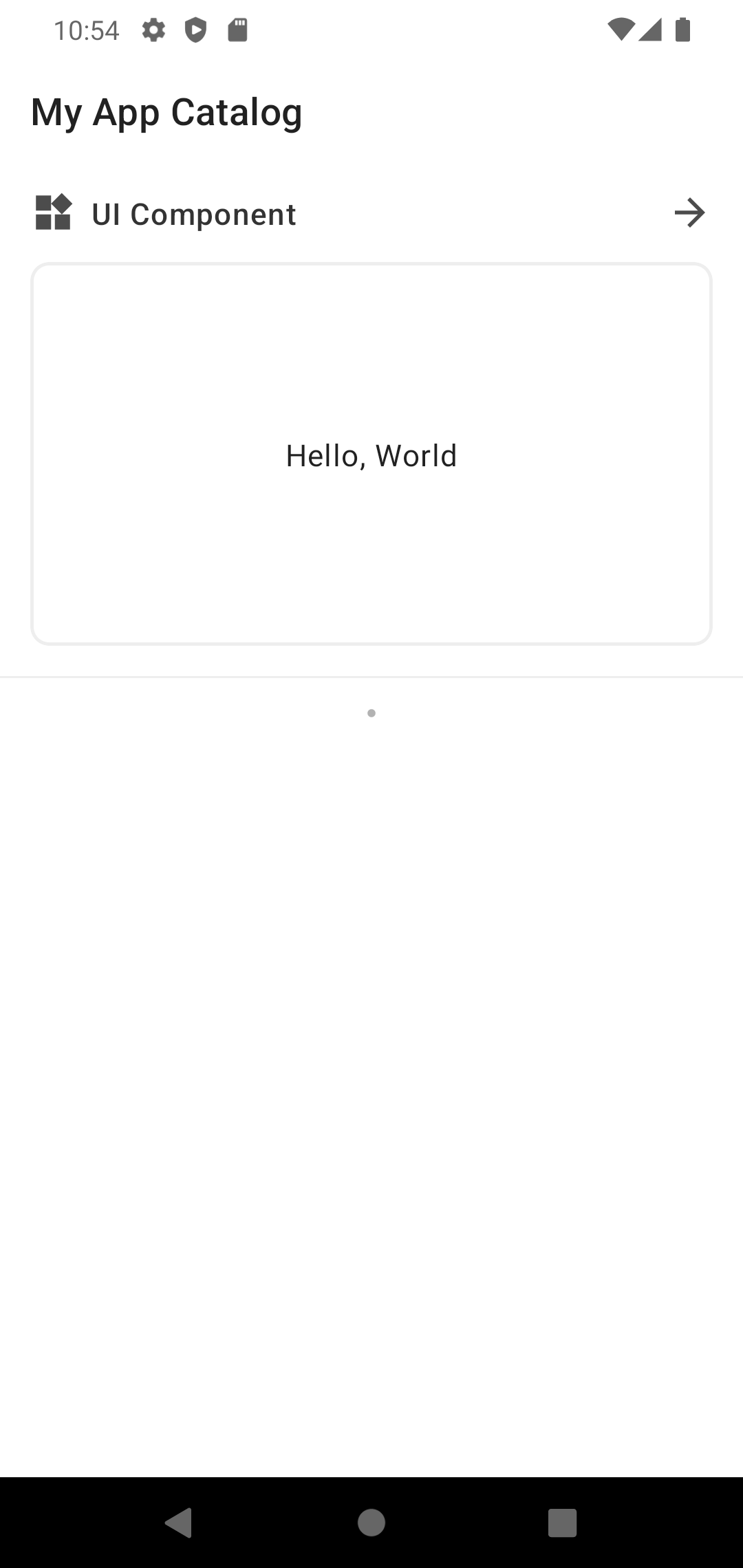
You can use the group
function to group components.
registerKatalog(
title = "My App Catalog"
) {
group("Group 1") {
compose("UI Component") {
/* ... */
}
}
group("Group 2") {
/* ... */
}
}
The group
can also be assigned to a variable.
val group1 = group("Group 1") {
/* ... */
}
val group2 = group("Group 2") {
/* ... */
}
registerKatalog {
title = "My App Catalog"
group(group1, group2)
}
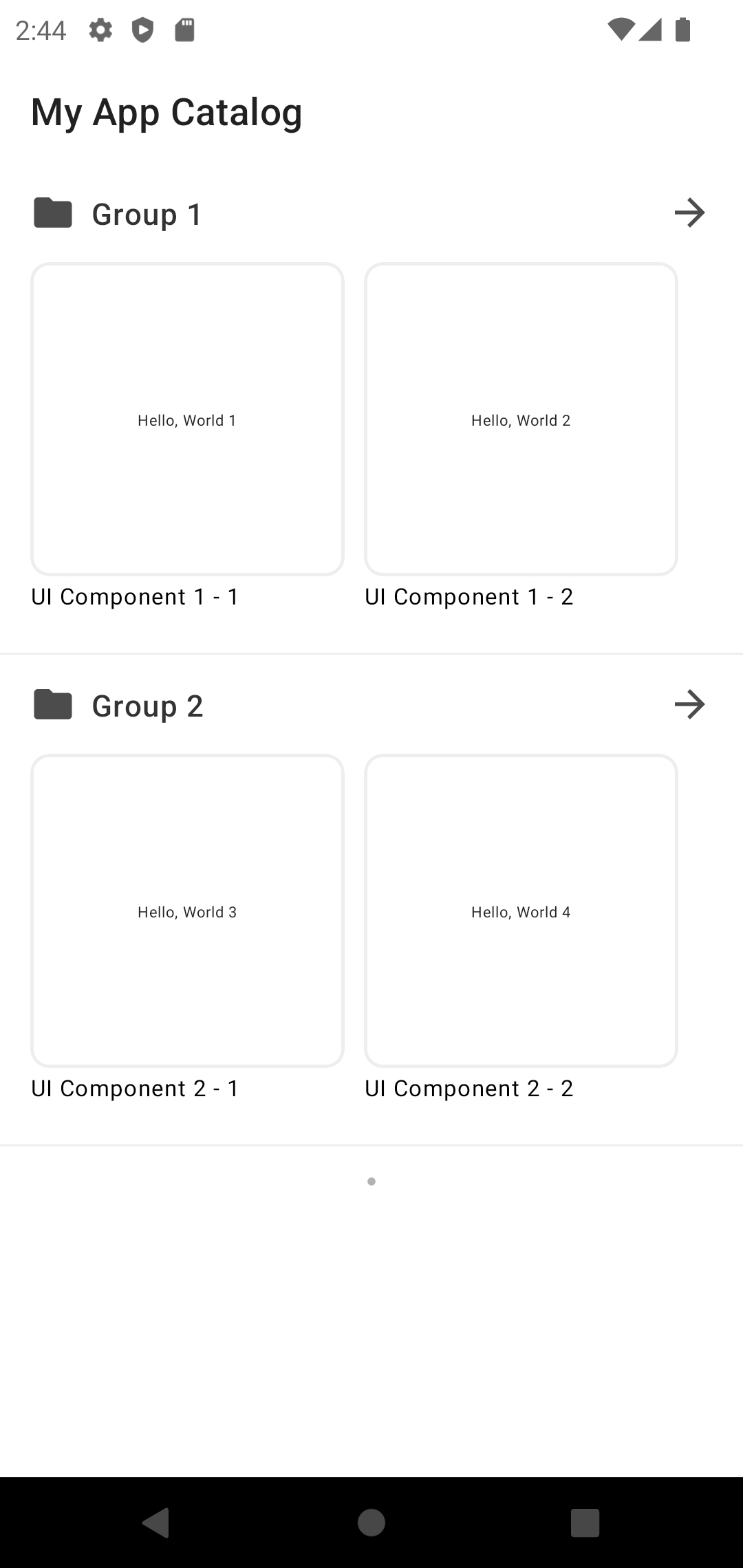
step3: Start Catalog Activity
Start KatalogActivity
from your debug menu.
KatalogActivity.start(activity)